As far as I had to fix small project I had a task to fix JSF application. I need:
1.Add sorting
2.Fix flow
What I have is ant with many libs and JSF version 1.1.2
Problems:
1.JSF doesn't support table sorting.So do use this I need to use extensions such as Tomahawk or Trinidad as far as I worked with Tomahawk I used it.Last version in maven repositary is Tomahawk 1.1.5 so I used it. JSF 1.1.2 is not compatible with it so I replaced it with myfaces 1.1.5.After that many operations in applications didn't worked I had a lot of time to fix
In the same time I started to write the same application but on GWT. The 1 problem was that JSF Bean has UI, SQL, POJO in 1 Bean.
Time estimations will be discuss later
Thursday, January 15, 2009
Hibernate Filters
Task:
Show feed executors with specified date

So Feed Entity:
Feed Runner Entity
Show feed executors with specified date
Libraries:
org.hibernate
hibernate-annotations
3.3.1.GA
org.hibernate
hibernate-commons-annotations
3.3.0.ga
So Feed Entity:
@Entity(name="FEED")
@FilterDef(name="status", parameters=@ParamDef( name="date", type="date" ) )
public class Feed {
@Id
@Column(name="FEED_ID")
private int id;
@Column(name="NAME")
private String name;
@OneToMany(fetch=FetchType.EAGER)
@JoinColumn(name = "FEED_ID")
@BatchSize(size=50)
@Fetch(FetchMode.SELECT)
@Filter(name="status", condition="BUSINESS_DATE=(select max(x.business_date) from FEED_RUN x where x.BUSINESS_DATE <= :date AND FEED_ID=x.FEED_ID)")
private List feedExecution;
}
Feed Runner Entity
@Entity(name = "FEED_RUN")
@Where(clause = "STATUS_ID <> 4")
public class FeedExecution {
@Id
@Column(name = "FEED_RUN_ID")
private int id;
@Column(name = "BUSINESS_DATE")
@Temporal(TemporalType.DATE)
private Date businessDate;
@ManyToOne(fetch = FetchType.EAGER)
@JoinColumn(name = "STATUS_ID")
private Status status;
@Formula("decode((SELECT COUNT(*) FROM file_load fl WHERE feed_run_id = fl.feed_run_id)+(SELECT COUNT(*) FROM event_log ev WHERE feed_run_id = ev.feed_run_id)+(SELECT COUNT(*) FROM error_log er WHERE feed_run_id = er.feed_run_id), 0, 0, 1)")
private boolean executed;
}
Migration from JBoss 4.0 to Tomcat 6.0(JNDI context)
JNDI resources types:
1.Global - myDS
2.Local java:/comp/env/myDS
By default JBOSS has global JNDI context
Tomcat has local JNDI context
To export property:
Where propertyDS - name of system property that will be used in your application
1.Global - myDS
2.Local java:/comp/env/myDS
By default JBOSS has global JNDI context
Tomcat has local JNDI context
To export property:
JAVA_OPTS="$JAVA_OPTS -DpropertyDS=java:/comp/env/myDS"
Where propertyDS - name of system property that will be used in your application
Monday, January 5, 2009
Creating your own maven plugin
Maven plugin:
Project->New->Maven Project->Next then select:
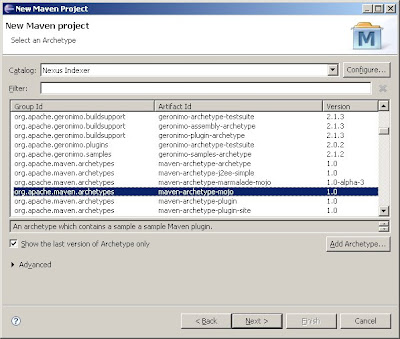
Your Mojo class will look like:
Then you can run it via command line:
mvn groupId:artifactId:version:goal
In this case go to your project directory
Project->New->Maven Project->Next then select:
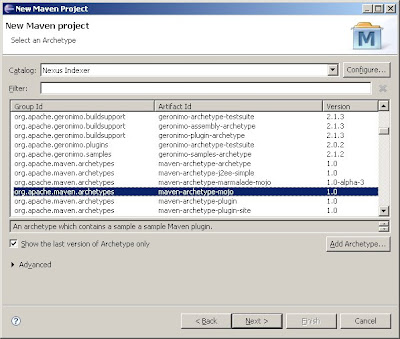
Your Mojo class will look like:
import org.apache.maven.plugin.AbstractMojo;This plugin just creates file. Pay attention that comments are really a part of function.
import org.apache.maven.plugin.MojoExecutionException;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
/**
* Goal which touches a timestamp file.
*
* @goal ddw
*
* @phase process-sources
*/
public class MyMojo extends AbstractMojo {
/**
* Location of the file.
* expression says which parameter to use
* @parameter expression="${outputDirectory}"
* @required
*/
private File outputDirectory;
public void execute() throws MojoExecutionException {
File f = outputDirectory;
if (!f.exists()) {
f.mkdirs();
}
File touch = new File(f, "touch.txt");
FileWriter w = null;
try {
w = new FileWriter(touch);
w.write("touch.txt");
} catch (IOException e) {
throw new MojoExecutionException("Error creating file " + touch, e);
} finally {
if (w != null) {
try {
w.close();
} catch (IOException e) {
// ignore
}
}
}
}
}
Then you can run it via command line:
mvn groupId:artifactId:version:goal
In this case go to your project directory
mvn com.ddw.plugin:ddw-plugin:0.0.1-SNAPSHOT:ddw -DoutputDirectory="C:\tmp"
Subscribe to:
Posts (Atom)