org.slf4j
slf4j-log4j12
1.5.8
Wednesday, December 23, 2009
SLF4J
SLF4J-simple can't be configured via log4j.properties. To configure it use slf4j-log4j12
Tuesday, November 10, 2009
WINDOW resize handler
public class XXX implements ResizeHandler{
public XXX(){
Window.addResizeHandler(this);
}
@Override
public void onResize(ResizeEvent event) {
//TODO YOUR CODE AFTER RESIZING
}
}
public XXX(){
Window.addResizeHandler(this);
}
@Override
public void onResize(ResizeEvent event) {
//TODO YOUR CODE AFTER RESIZING
}
}
Tuesday, September 29, 2009
Date Formatter
Is the ParseException happens?
SimpleDateFormat df = new SimpleDateFormat("dd-MM-yyyy");
Date date = null;
try {
//1
date = df.parse("12-34-2007");
} catch (ParseException e) {
System.out.println("Wrong date");
}
System.out.println(date.toString());
Actually not. To prevent this add to //1
df.setLenient(false);
Letient parameter is true by default that's why to match format strictly need
setting parameter to false
SimpleDateFormat df = new SimpleDateFormat("dd-MM-yyyy");
Date date = null;
try {
//1
date = df.parse("12-34-2007");
} catch (ParseException e) {
System.out.println("Wrong date");
}
System.out.println(date.toString());
Actually not. To prevent this add to //1
df.setLenient(false);
Letient parameter is true by default that's why to match format strictly need
setting parameter to false
Sunday, September 20, 2009
Monday, May 25, 2009
Converting image
As our Designer was fired because of crisis - I had to change images size. So imagemagic is excelent for this use.
mogrify -gravity Center -crop 30X22+0+0 *.png
mogrify -format png *.jpg
mogrify -resize 30x22+0+0 *.*
mogrify -resize 120% *.*
identify -format "%wx%h \n" *.*
Friday, May 8, 2009
Hibernate Custom Type
Database has for example wrong state and can't save 1 or 0, 'Y' or 'N', 'YES' or 'NO'
So you need to save state as 1 and 2. Seems that database need refactoring... yes you are right but if you can't? Here is decision - custom type
And It's usage
So you need to save state as 1 and 2. Seems that database need refactoring... yes you are right but if you can't? Here is decision - custom type
package com.types;
import java.io.Serializable;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Types;
import org.hibernate.HibernateException;
import org.hibernate.usertype.UserType;
public class OneTwoBooleanType implements UserType{
private final static int DB_TRUE = 1;
private final static int DB_FALSE = 2;
public Object assemble(Serializable cached, Object owner) throws HibernateException {
return cached;
}
public Object deepCopy(Object value) throws HibernateException {
return value;
}
public Serializable disassemble(Object value) throws HibernateException {
return (Serializable) value;
}
public boolean equals(Object x, Object y) throws HibernateException {
if(x==null){
return false;
}
return ((Boolean)x).equals(y);
}
public int hashCode(Object x) throws HibernateException {
return x.hashCode();
}
public boolean isMutable() {
return false;
}
public Object nullSafeGet(ResultSet rs, String[] names, Object owner) throws HibernateException, SQLException {
Number val = (Number) rs.getObject(names[0]);
if(val==null){
return false;
}
return val.intValue() == DB_TRUE ? true : false;
}
public void nullSafeSet(PreparedStatement st, Object value, int index) throws HibernateException, SQLException {
int dbValue;
if(value==null){
dbValue = DB_FALSE;
} else {
dbValue = (Boolean) value ? DB_TRUE : DB_FALSE;
}
st.setInt(index, dbValue);
}
public Object replace(Object original, Object target, Object owner) throws HibernateException {
return original;
}
public Class returnedClass() {
return Boolean.class;
}
public int[] sqlTypes() {
return new int[]{Types.SMALLINT};
}
}
And It's usage
@Column(name="INTERNAL_STATUS_ID")
@Type(type="com.types.OneTwoBooleanType")
private boolean isVisible;
Tuesday, April 21, 2009
Substract queries
If you need to check differences between tables use this query:
select * from t1
minus
select * from t2
Tuesday, April 7, 2009
Java version number
import java.io.DataInputStream;
import java.io.FileInputStream;
import java.io.IOException;
public class ClassVersionChecker {
public static void main(String[] args) throws IOException {
for (int i = 0; i < args.length; i++)
checkClassVersion(args[i]);
}
private static void checkClassVersion(String filename) throws IOException {
DataInputStream in = new DataInputStream(new FileInputStream(filename));
int magic = in.readInt();
if (magic != 0xcafebabe) {
System.out.println(filename + " is not a valid class!");
}
int minor = in.readUnsignedShort();
int major = in.readUnsignedShort();
System.out.println(filename + ": " + major + " . " + minor);
in.close();
}
}
1.Compile
javac ClassVersionChecker.java
2.Execute in unix console
find . -name *.class|xargs java -cp /c/tmp ClassVersionChecker|grep 50
where last number is class version
major minor Java platform version
45 3 1.0
45 3 1.1
46 0 1.2
47 0 1.3
48 0 1.4
49 0 1.5
50 0 1.6
Friday, March 27, 2009
Statistical SQL query
For example you need to count how many companies in which source system:
select count(*), source_system_id from company group by rollup(source_system_id)
Thursday, January 15, 2009
Migration from JSF to GWT 1.5
As far as I had to fix small project I had a task to fix JSF application. I need:
1.Add sorting
2.Fix flow
What I have is ant with many libs and JSF version 1.1.2
Problems:
1.JSF doesn't support table sorting.So do use this I need to use extensions such as Tomahawk or Trinidad as far as I worked with Tomahawk I used it.Last version in maven repositary is Tomahawk 1.1.5 so I used it. JSF 1.1.2 is not compatible with it so I replaced it with myfaces 1.1.5.After that many operations in applications didn't worked I had a lot of time to fix
In the same time I started to write the same application but on GWT. The 1 problem was that JSF Bean has UI, SQL, POJO in 1 Bean.
Time estimations will be discuss later
1.Add sorting
2.Fix flow
What I have is ant with many libs and JSF version 1.1.2
Problems:
1.JSF doesn't support table sorting.So do use this I need to use extensions such as Tomahawk or Trinidad as far as I worked with Tomahawk I used it.Last version in maven repositary is Tomahawk 1.1.5 so I used it. JSF 1.1.2 is not compatible with it so I replaced it with myfaces 1.1.5.After that many operations in applications didn't worked I had a lot of time to fix
In the same time I started to write the same application but on GWT. The 1 problem was that JSF Bean has UI, SQL, POJO in 1 Bean.
Time estimations will be discuss later
Hibernate Filters
Task:
Show feed executors with specified date

So Feed Entity:
Feed Runner Entity
Show feed executors with specified date
Libraries:
org.hibernate
hibernate-annotations
3.3.1.GA
org.hibernate
hibernate-commons-annotations
3.3.0.ga
So Feed Entity:
@Entity(name="FEED")
@FilterDef(name="status", parameters=@ParamDef( name="date", type="date" ) )
public class Feed {
@Id
@Column(name="FEED_ID")
private int id;
@Column(name="NAME")
private String name;
@OneToMany(fetch=FetchType.EAGER)
@JoinColumn(name = "FEED_ID")
@BatchSize(size=50)
@Fetch(FetchMode.SELECT)
@Filter(name="status", condition="BUSINESS_DATE=(select max(x.business_date) from FEED_RUN x where x.BUSINESS_DATE <= :date AND FEED_ID=x.FEED_ID)")
private List feedExecution;
}
Feed Runner Entity
@Entity(name = "FEED_RUN")
@Where(clause = "STATUS_ID <> 4")
public class FeedExecution {
@Id
@Column(name = "FEED_RUN_ID")
private int id;
@Column(name = "BUSINESS_DATE")
@Temporal(TemporalType.DATE)
private Date businessDate;
@ManyToOne(fetch = FetchType.EAGER)
@JoinColumn(name = "STATUS_ID")
private Status status;
@Formula("decode((SELECT COUNT(*) FROM file_load fl WHERE feed_run_id = fl.feed_run_id)+(SELECT COUNT(*) FROM event_log ev WHERE feed_run_id = ev.feed_run_id)+(SELECT COUNT(*) FROM error_log er WHERE feed_run_id = er.feed_run_id), 0, 0, 1)")
private boolean executed;
}
Migration from JBoss 4.0 to Tomcat 6.0(JNDI context)
JNDI resources types:
1.Global - myDS
2.Local java:/comp/env/myDS
By default JBOSS has global JNDI context
Tomcat has local JNDI context
To export property:
Where propertyDS - name of system property that will be used in your application
1.Global - myDS
2.Local java:/comp/env/myDS
By default JBOSS has global JNDI context
Tomcat has local JNDI context
To export property:
JAVA_OPTS="$JAVA_OPTS -DpropertyDS=java:/comp/env/myDS"
Where propertyDS - name of system property that will be used in your application
Monday, January 5, 2009
Creating your own maven plugin
Maven plugin:
Project->New->Maven Project->Next then select:
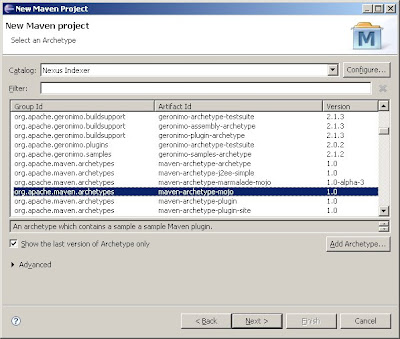
Your Mojo class will look like:
Then you can run it via command line:
mvn groupId:artifactId:version:goal
In this case go to your project directory
Project->New->Maven Project->Next then select:
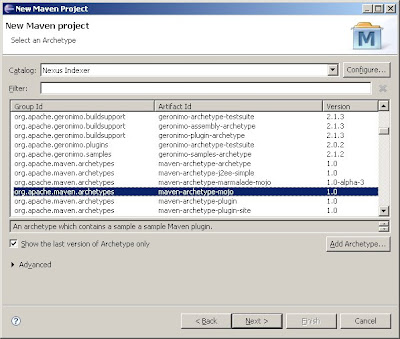
Your Mojo class will look like:
import org.apache.maven.plugin.AbstractMojo;This plugin just creates file. Pay attention that comments are really a part of function.
import org.apache.maven.plugin.MojoExecutionException;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
/**
* Goal which touches a timestamp file.
*
* @goal ddw
*
* @phase process-sources
*/
public class MyMojo extends AbstractMojo {
/**
* Location of the file.
* expression says which parameter to use
* @parameter expression="${outputDirectory}"
* @required
*/
private File outputDirectory;
public void execute() throws MojoExecutionException {
File f = outputDirectory;
if (!f.exists()) {
f.mkdirs();
}
File touch = new File(f, "touch.txt");
FileWriter w = null;
try {
w = new FileWriter(touch);
w.write("touch.txt");
} catch (IOException e) {
throw new MojoExecutionException("Error creating file " + touch, e);
} finally {
if (w != null) {
try {
w.close();
} catch (IOException e) {
// ignore
}
}
}
}
}
Then you can run it via command line:
mvn groupId:artifactId:version:goal
In this case go to your project directory
mvn com.ddw.plugin:ddw-plugin:0.0.1-SNAPSHOT:ddw -DoutputDirectory="C:\tmp"
Subscribe to:
Posts (Atom)